2/24/2023
The Why
Hiding tabs in a Model Driven app until a record is created can simplify the interface and reduce clutter. If certain tabs are not relevant until a record is created, it can be helpful to hide them until they are needed. This can make the app more intuitive and easier to use for the end user.
Additionally, hiding tabs can guide the user through the app, ensuring that they are only presented with options relevant to their current task. This can improve the overall user experience and increase satisfaction.
The How
Expanding on the previous writeup, we will now hide all tabs that the user does not need to see until after the record is created. We will be hiding the Supervisor, Higher Approval, and Documentation Tab until the record is created. Additionally,we will be hiding the Higher Approval tab if the cost is ≤ $100.

Code
The first part of the code declares the function and the formcontext variable
function onFormLoad(executionContext) {
var formContext = executionContext.getFormContext();
The next part gets the record id
// Get the record ID
var recordId = formContext.data.entity.getId();
Next, we check if the record exists. If it does not exist, the tabs will be hidden.
// Check if the record has been created
if (recordId === null || recordId === "") {
// If the record has not been created, hide the tabs
formContext.ui.tabs.get("tab_documentation").setVisible(false);
formContext.ui.tabs.get("tab_sup").setVisible(false);
formContext.ui.tabs.get("tab_higherapproval").setVisible(false);
} else {
// If the record has been created, show all tabs
formContext.ui.tabs.get("tab_documentation").setVisible(true);
formContext.ui.tabs.get("tab_sup").setVisible(true);
formContext.ui.tabs.get("tab_higherapproval").setVisible(true);
Lastly we check to see if the cost field is ≤ $100
// Check the cost field value
var costField = formContext.getAttribute("eddev_cost");
if (costField.getValue() <= 100) {
// If the cost is less than or equal to 100, hide the tab_higherapproval
formContext.ui.tabs.get("tab_higherapproval").setVisible(false);
All put together.
function onFormLoad(executionContext) {
var formContext = executionContext.getFormContext();
// Get the record ID
var recordId = formContext.data.entity.getId();
// Check if the record has been created
if (recordId === null || recordId === "") {
// If the record has not been created, hide the tabs
formContext.ui.tabs.get("tab_documentation").setVisible(false);
formContext.ui.tabs.get("tab_sup").setVisible(false);
formContext.ui.tabs.get("tab_higherapproval").setVisible(false);
} else {
// If the record has been created, show all tabs
formContext.ui.tabs.get("tab_documentation").setVisible(true);
formContext.ui.tabs.get("tab_sup").setVisible(true);
formContext.ui.tabs.get("tab_higherapproval").setVisible(true);
// Check the cost field value
var costField = formContext.getAttribute("eddev_cost");
if (costField.getValue() <= 100) {
// If the cost is less than or equal to 100, hide the tab_higherapproval
formContext.ui.tabs.get("tab_higherapproval").setVisible(false);
}
}
}
Updating WebPart
As we are using the same .js file, we can simply update the one that was created before.
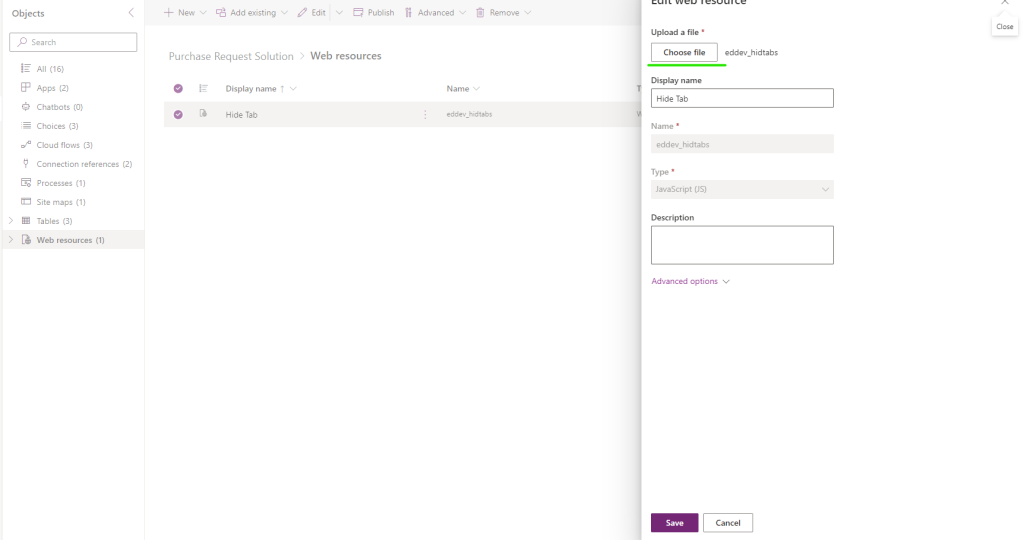
Form
Change the function to "onFormLoad" on the form's On Load event.
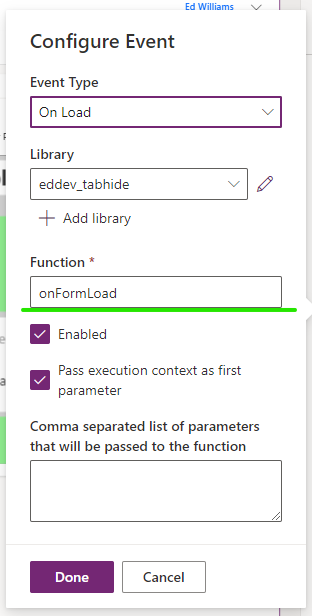
Change the function in the cost field to "onFormLoad".
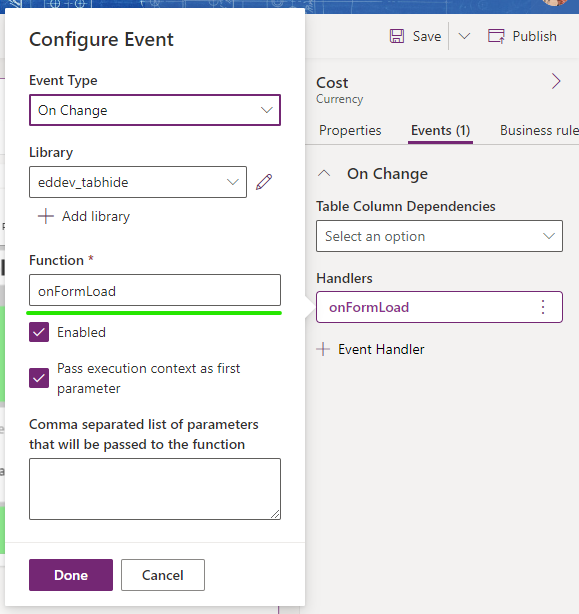
Save and Publish the form.